バイト
日本語 | バイト |
英語 | byte |
ふりがな | ばいと |
フリガナ | バイト |
コンピューターにおける情報の単位のひとつ。
1バイトは8ビットである。
つまりビットが8個並んでいたらそれが1バイトである。
たとえば、int型変数のサイズは32ビット=4バイトのサイズということになる。
また、16進数1桁は4ビットなので、1バイトは16進数で2桁となり、1バイトを16進数2桁で表現することが多い。
1バイトは2の8乗、つまり256種類の表現が可能である。
プリミティブ型に、この1バイト分のデータを格納できるbyte型が存在する。
byte型の変数はメモリ上に8ビットの領域を確保し、-128~127までの整数値を格納することができる。
つまり文字通り、1バイト分のデータを格納できる。
そのため、バイト単位の操作を行う際にこの型を使用することが多い。
Javaでは、1バイトは8ビットである。
だが、1バイトが8ビットではないプラットフォームも存在する。
バイトは本来「ひとかたまりのビット」という意味である。そしてそのビットの数は、コンピューターやOSによって異なる。最近のものはほとんどが8ビットだが、昔のものは5ビットや7ビットのものもある。
ネットワークを通して他のコンピューターにデータを送信する際に、1バイトが8ビットではないコンピューターやOSに送信すると問題が発生する可能性があるため、珍しいコンピューターやOSの場合には確認した方がいいだろう。
1バイトは8ビットである。
つまりビットが8個並んでいたらそれが1バイトである。
たとえば、int型変数のサイズは32ビット=4バイトのサイズということになる。
また、16進数1桁は4ビットなので、1バイトは16進数で2桁となり、1バイトを16進数2桁で表現することが多い。
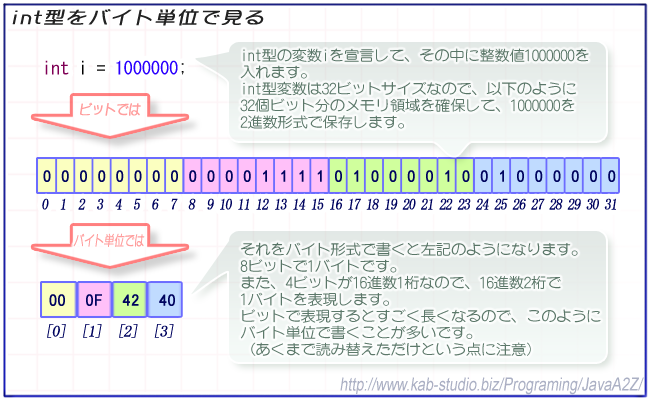
1バイトは2の8乗、つまり256種類の表現が可能である。
プリミティブ型に、この1バイト分のデータを格納できるbyte型が存在する。
byte型の変数はメモリ上に8ビットの領域を確保し、-128~127までの整数値を格納することができる。
つまり文字通り、1バイト分のデータを格納できる。
そのため、バイト単位の操作を行う際にこの型を使用することが多い。
Javaでは、1バイトは8ビットである。
だが、1バイトが8ビットではないプラットフォームも存在する。
バイトは本来「ひとかたまりのビット」という意味である。そしてそのビットの数は、コンピューターやOSによって異なる。最近のものはほとんどが8ビットだが、昔のものは5ビットや7ビットのものもある。
ネットワークを通して他のコンピューターにデータを送信する際に、1バイトが8ビットではないコンピューターやOSに送信すると問題が発生する可能性があるため、珍しいコンピューターやOSの場合には確認した方がいいだろう。
参考サイト
- (参考サイトはありません)
// Sample.java
public class Sample
{
public static void main( String[] args )
{
// int型の変数を宣言します。
int i;
// メモリ上に32ビットの領域が確保されました。
// ここに整数値1000000を格納します。
i = 1000000;
// それを出力します。
System.out.println( i );
// 1000000
// int型変数に格納された「1000000」がどのように
// ビットとして格納されているか見てみます。
outputIntBit( i );
// 00000000 00001111 01000010 01000000
// このように、「1000000」というint型整数は、
// 「00000000 00001111 01000010 01000000」という
// 「0か1」を32個並べた形で格納されています。
// で、このスペースで区切った、8ビットずつの塊が
// 「バイト」です。
// int型変数は32ビットなので4バイトということに
// なり、それぞれのバイトは
// 「00000000」「00001111」「01000010」「01000000」
// となるわけです。
// ただ、8ビットをそのまま書くと長くなるので、
// 16進数を使って表記することが多いです。
// 16進数1桁は4ビットなので、1バイトは16進数2桁で
// 表現できます。
outputIntByte( i );
// 00 0f 42 40
}
/**
* int型変数の中身をビット形式で出力します。
*/
private static void outputIntBit( int i )
{
// int型変数をビット形式で文字列化します。
String source = Integer.toBinaryString( i );
// 左0埋めします。
StringBuffer strbuf = new StringBuffer();
for( int iF1 = source.length(); iF1 < 32; ++iF1 )
{
strbuf.append( "0" );
}
strbuf.append( source );
// バイト単位になるようスペースを入れます。
strbuf.insert( 24, " " );
strbuf.insert( 16, " " );
strbuf.insert( 8, " " );
System.out.println( strbuf.toString() );
}
/**
* int型変数の中身をバイト形式で出力します。
*/
private static void outputIntByte( int i )
{
// int型変数を16進数形式で文字列化します。
String source = Integer.toHexString( i );
// 左0埋めします。
StringBuffer strbuf = new StringBuffer();
for( int iF1 = source.length(); iF1 < 8; ++iF1 )
{
strbuf.append( "0" );
}
strbuf.append( source );
// バイト単位になるようスペースを入れます。
strbuf.insert( 6, " " );
strbuf.insert( 4, " " );
strbuf.insert( 2, " " );
System.out.println( strbuf.toString() );
}
}
public class Sample
{
public static void main( String[] args )
{
// int型の変数を宣言します。
int i;
// メモリ上に32ビットの領域が確保されました。
// ここに整数値1000000を格納します。
i = 1000000;
// それを出力します。
System.out.println( i );
// 1000000
// int型変数に格納された「1000000」がどのように
// ビットとして格納されているか見てみます。
outputIntBit( i );
// 00000000 00001111 01000010 01000000
// このように、「1000000」というint型整数は、
// 「00000000 00001111 01000010 01000000」という
// 「0か1」を32個並べた形で格納されています。
// で、このスペースで区切った、8ビットずつの塊が
// 「バイト」です。
// int型変数は32ビットなので4バイトということに
// なり、それぞれのバイトは
// 「00000000」「00001111」「01000010」「01000000」
// となるわけです。
// ただ、8ビットをそのまま書くと長くなるので、
// 16進数を使って表記することが多いです。
// 16進数1桁は4ビットなので、1バイトは16進数2桁で
// 表現できます。
outputIntByte( i );
// 00 0f 42 40
}
/**
* int型変数の中身をビット形式で出力します。
*/
private static void outputIntBit( int i )
{
// int型変数をビット形式で文字列化します。
String source = Integer.toBinaryString( i );
// 左0埋めします。
StringBuffer strbuf = new StringBuffer();
for( int iF1 = source.length(); iF1 < 32; ++iF1 )
{
strbuf.append( "0" );
}
strbuf.append( source );
// バイト単位になるようスペースを入れます。
strbuf.insert( 24, " " );
strbuf.insert( 16, " " );
strbuf.insert( 8, " " );
System.out.println( strbuf.toString() );
}
/**
* int型変数の中身をバイト形式で出力します。
*/
private static void outputIntByte( int i )
{
// int型変数を16進数形式で文字列化します。
String source = Integer.toHexString( i );
// 左0埋めします。
StringBuffer strbuf = new StringBuffer();
for( int iF1 = source.length(); iF1 < 8; ++iF1 )
{
strbuf.append( "0" );
}
strbuf.append( source );
// バイト単位になるようスペースを入れます。
strbuf.insert( 6, " " );
strbuf.insert( 4, " " );
strbuf.insert( 2, " " );
System.out.println( strbuf.toString() );
}
}
// Sample.java public class Sample { public static void main( String[] args ) { // int型の変数を宣言します。 int i; // メモリ上に32ビットの領域が確保されました。 // ここに整数値1000000を格納します。 i = 1000000; // それを出力します。 System.out.println( i ); // 1000000 // int型変数に格納された「1000000」がどのように // ビットとして格納されているか見てみます。 outputIntBit( i ); // 00000000 00001111 01000010 01000000 // このように、「1000000」というint型整数は、 // 「00000000 00001111 01000010 01000000」という // 「0か1」を32個並べた形で格納されています。 // で、このスペースで区切った、8ビットずつの塊が // 「バイト」です。 // int型変数は32ビットなので4バイトということに // なり、それぞれのバイトは // 「00000000」「00001111」「01000010」「01000000」 // となるわけです。 // ただ、8ビットをそのまま書くと長くなるので、 // 16進数を使って表記することが多いです。 // 16進数1桁は4ビットなので、1バイトは16進数2桁で // 表現できます。 outputIntByte( i ); // 00 0f 42 40 } /** * int型変数の中身をビット形式で出力します。 */ private static void outputIntBit( int i ) { // int型変数をビット形式で文字列化します。 String source = Integer.toBinaryString( i ); // 左0埋めします。 StringBuffer strbuf = new StringBuffer(); for( int iF1 = source.length(); iF1 < 32; ++iF1 ) { strbuf.append( "0" ); } strbuf.append( source ); // バイト単位になるようスペースを入れます。 strbuf.insert( 24, " " ); strbuf.insert( 16, " " ); strbuf.insert( 8, " " ); System.out.println( strbuf.toString() ); } /** * int型変数の中身をバイト形式で出力します。 */ private static void outputIntByte( int i ) { // int型変数を16進数形式で文字列化します。 String source = Integer.toHexString( i ); // 左0埋めします。 StringBuffer strbuf = new StringBuffer(); for( int iF1 = source.length(); iF1 < 8; ++iF1 ) { strbuf.append( "0" ); } strbuf.append( source ); // バイト単位になるようスペースを入れます。 strbuf.insert( 6, " " ); strbuf.insert( 4, " " ); strbuf.insert( 2, " " ); System.out.println( strbuf.toString() ); } }
「みだし」に含まれているページ
「解説」に含まれているページ
- 16進数
- BufferedInputStream
- BufferedOutputStream
- byte
- ByteArrayInputStream
- ByteArrayOutputStream
- C++言語
- char
- CharArrayWriter
- Cookie
- DataInputStream
- DataOutputStream
- DOM
- double
- EUC
- FileInputStream
- FileOutputStream
- FileReader
- FilterInputStream
- FilterOutputStream
- FilterWriter
- float
- getBytes
- InputStream
- InputStreamReader
- int
- IPアドレス
- ISO-2022-JP
- LineNumberInputStream
- long
- ObjectInputStream
- ObjectOutputStream
- OutputStream
- OutputStreamWriter
- PipedInputStream
- PipedOutputStream
- PrintStream
- PrintWriter
- PushbackInputStream
- Reader
- Rss4j
- SequenceInputStream
- short
- size
- StackOverflowError
- StringBufferInputStream
- StringWriter
- Unicode
- unsigned
- UTF-8
- Writer
- アドレス
- クッキー
- シフトJIS
- スタック領域
- ストリーム
- ソースプログラム
- バイト
- バイトコード
- バイトストリーム
- バイト入力ストリーム
- バイト出力ストリーム
- バイナリーファイル
- ビット
- ファイル
- フラッシュ
- プリミティブ型
- 全角
- 半角
- 半角カタカナ
- 文字
- 文字ストリーム
- 文字入力ストリーム
- 文字出力ストリーム
- 文字化け
「サンプルプログラムとか」に含まれているページ
- BufferedInputStream
- BufferedOutputStream
- BufferedReader
- BufferedWriter
- ByteArrayInputStream
- ByteArrayOutputStream
- char
- CharArrayReader
- DataInputStream
- DataOutputStream
- FileInputStream
- FileOutputStream
- FileReader
- FilterInputStream
- FilterOutputStream
- getBytes
- InputStream
- InputStreamReader
- ISO-8859-1
- Java仮想マシン
- LineNumberInputStream
- ObjectInputStream
- ObjectOutputStream
- OutputStream
- OutputStreamWriter
- PipedInputStream
- PipedOutputStream
- PipedReader
- PipedWriter
- PrintStream
- PushbackInputStream
- PushbackReader
- Reader
- SequenceInputStream
- StringBufferInputStream
- StringReader
- UDP
- バイト
- バイトコード
- バイトストリーム
- バイナリーファイル
- ブロックします
- 全角
- 半角
- 半角カタカナ
- 改行文字
- 文字
- 文字コード
- 標準入出力
- 環境変数